How to access the .txt file and Image file
create your xml file with an image and text with the position where you want .
copy your text file and image in your assets folder here I've pasted "text.txt" and "risk.jpg" in assets folder.
In the Textfile write your content as your own .Ex: Here I've written "Hi! This's Rakesh"
In your Java file ,use the snippets given below ,
package com.example.accessfromassets;
import java.io.IOException;
import java.io.InputStream;
import android.app.Activity;
import android.graphics.drawable.Drawable;
import android.os.Bundle;
import android.widget.ImageView;
import android.widget.TextView;
public class MainActivity extends Activity {
ImageView mImage;
TextView mText;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mImage = (ImageView)findViewById(R.id.imageView1);
mText = (TextView)findViewById(R.id.textView1);
loadDataFromAsset();
}
public void loadDataFromAsset() {
// load text
try {
// get input stream for text
InputStream is = getAssets().open("text.txt");
// check size
int size = is.available();
// create buffer for IO
byte[] buffer = new byte[size];
// get data to buffer
is.read(buffer);
// close stream
is.close();
// set result to TextView
mText.setText(new String(buffer));
}
catch (IOException ex) {
return;
}
// load image
try {
// get input stream
InputStream ims = getAssets().open("risk.jpg");
// load image as Drawable
Drawable d = Drawable.createFromStream(ims, null);
// set image to ImageView
mImage.setImageDrawable(d);
}
catch(IOException ex) {
return;
}
}
}
The Result will be ,
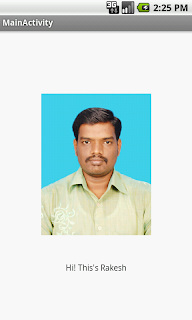
copy your text file and image in your assets folder here I've pasted "text.txt" and "risk.jpg" in assets folder.
In the Textfile write your content as your own .Ex: Here I've written "Hi! This's Rakesh"
In your Java file ,use the snippets given below ,
package com.example.accessfromassets;
import java.io.IOException;
import java.io.InputStream;
import android.app.Activity;
import android.graphics.drawable.Drawable;
import android.os.Bundle;
import android.widget.ImageView;
import android.widget.TextView;
public class MainActivity extends Activity {
ImageView mImage;
TextView mText;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mImage = (ImageView)findViewById(R.id.imageView1);
mText = (TextView)findViewById(R.id.textView1);
loadDataFromAsset();
}
public void loadDataFromAsset() {
// load text
try {
// get input stream for text
InputStream is = getAssets().open("text.txt");
// check size
int size = is.available();
// create buffer for IO
byte[] buffer = new byte[size];
// get data to buffer
is.read(buffer);
// close stream
is.close();
// set result to TextView
mText.setText(new String(buffer));
}
catch (IOException ex) {
return;
}
// load image
try {
// get input stream
InputStream ims = getAssets().open("risk.jpg");
// load image as Drawable
Drawable d = Drawable.createFromStream(ims, null);
// set image to ImageView
mImage.setImageDrawable(d);
}
catch(IOException ex) {
return;
}
}
}
The Result will be ,
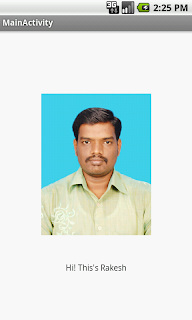
Comments
Post a Comment